Vue JS is a versatile and performant web framework that allows developers to build small or sophisticated web applications. Paired with the Vue CLI, Vuex, and Vue router, Vue is more than capable of handling a production-level environment.
Out of the box, Vue presents some pretty impressive numbers, but of course, as you reach a certain point in development the speed of your application may decrease and you may notice things seem a bit slower than expected. Cherry-picking and optimizing imports is a great way to decrease bundle size and speed up page loads.
Cherry Picking
When using a module bundler you can optionally import only specific components and directives. Pulling in a specific component or set of components only when needed can greatly decrease the size of your bundle.
Let’s use Bootstrap-Vue as an example.
Registering and using Bootstrap-Vue is easy and allows for easy prototyping.
//main.js
import Vue from 'vue'
import { BootstrapVue, IconsPlugin } from 'bootstrap-vue'
// Import Bootstrap an BootstrapVue CSS files (order is important)
import 'bootstrap/dist/css/bootstrap.css'
import 'bootstrap-vue/dist/bootstrap-vue.css'
// Make BootstrapVue available throughout your project
Vue.use(BootstrapVue)
// Optionally install the BootstrapVue icon components plugin
Vue.use(IconsPlugin)
//Nav.vue
<template>
<div>
//Bootstrap-Vue Nav Component
<b-nav>
<b-nav-item active>Active</b-nav-item>
<b-nav-item>Link</b-nav-item>
<b-nav-item>Another Link</b-nav-item>
<b-nav-item disabled>Disabled</b-nav-item>
</b-nav>
</div>
</template>
export default {
name: 'Nav'
}
Quick and easy, right? Let’s check on our bundle size after including Bootstrap-Vue in our project.
157.98 KB gzipped after registering Bootstrap-Vue (101.87 KB gzipped). This is a pretty heavy addition when compared with our initial bundle size. Let’s see if we can bring this number down by cherry-picking the components we actually need, instead of importing all components from the package.
When using a module bundler you can optionally import only specific components and directives. Pulling in a specific component or set of components only when needed can greatly decrease the size of your bundle.
We will cherry-pick the “nav” component from the previous example.
//main.js
import Vue from 'vue'
import { NavPlugin } from 'bootstrap-vue'
// Import Bootstrap an BootstrapVue CSS files (order is important)
import 'bootstrap/dist/css/bootstrap.css'
import 'bootstrap-vue/dist/bootstrap-vue.css'
// Make BootstrapVue available throughout your project
Vue.use(NavPlugin)
// Optionally install the BootstrapVue icon components plugin
Vue.use(IconsPlugin)
//Nav.vue
<template>
<div>
//Bootstrap-Vue Nav Component
<b-nav>
<b-nav-item active>Active</b-nav-item>
<b-nav-item>Link</b-nav-item>
<b-nav-item>Another Link</b-nav-item>
<b-nav-item disabled>Disabled</b-nav-item>
</b-nav>
</div>
</template>
import { BNav, BNavItem } from 'bootstrap-vue';
export default {
name: 'Nav',
components: {
BNav,
BNavItem
}
}
Let’s check on our bundle size after cherry-picking.
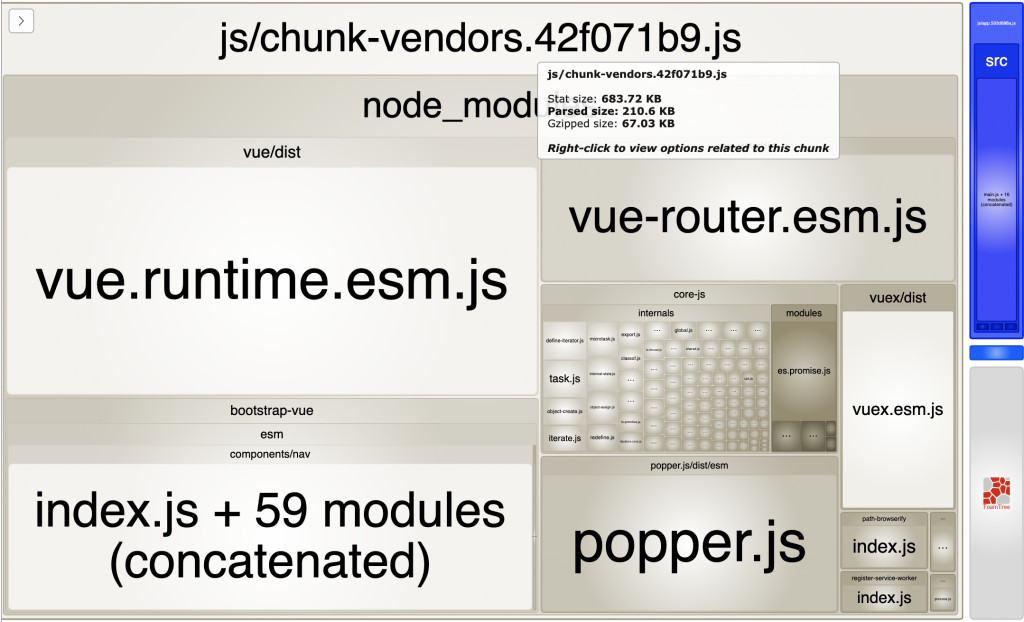
>50% decrease in our bundle size after cherry-picking. In a real-world application we would use more components, but even still, importing only what you need makes a huge difference. Utilizing modern-day best web practices such as cherry-picking, tree-shaking, and code splitting is just a couple of ways that could optimize your application and ultimately create a better experience for your users.